We can use Oracle cloud Object Storage to host a static website. Static web pages can contain client-side technologies such as HTML, CSS, and JavaScript. They cannot contain dynamic content such as server-side scripts like PHP, JSP or ASP.NET.
Step 1:
We need to create a public bucket and upload the static website contents. Once the bucket is created edit the visibility to give public access.
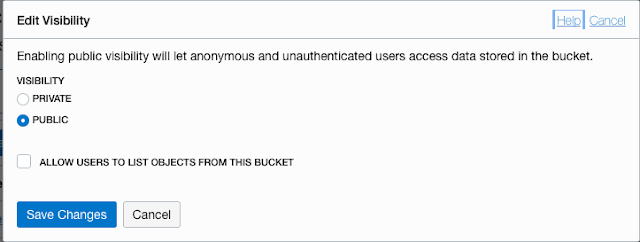
Step 2:
Upload the files, here is the trick. Object storage doesn't support the hierarchy of folder structure. So we have to name each file based on its path.
For example, we have a index.html that has a link to the file page-1.html inside the pages folder.
We have to upload index.html as index.html and page-1.html as "pages/page-1.html". So that links will work.
I know this can be tedious since a website contains hundreds of html files, images, css and javascript. So I have automated this process.
Upload Tool
The scripts are available at https://github.com/pallabrath/myexpjava/tree/master/oci-os-static-web-upload-util
It can be cloned by git or download as a zip (https://github.com/pallabrath/myexpjava) and then unzip
1. Move to the oci-os-static-web-upload-util directory.
2. To run the script we need node and npm to be installed in our environment.
Node - https://nodejs.org/en/
Npm - https://docs.npmjs.com/downloading-and-installing-node-js-and-npm
Npm - https://docs.npmjs.com/downloading-and-installing-node-js-and-npm
3. Required inputs for this tool need to be configured in upload-config.json.
{
"webdir" : "/Users/pallab/mylab/oci-os-static-web", # This is the path of the static web need to be uploaded
"index" : "index.html", # Its the index/home page of your website
"configurationFilePath" : "~/.oci/config", # OCI credential configuration
"configProfile" : "DEFAULT", # OCI credentail config profile
"comaprtmentOCId" : "ocid1.compartment.oc1......", # OCI compartment OCID where we want to upload
"bucketName" : "myexpdemo" # bucket name to be created
}
Details how to create OCI Credential configuration refer # https://docs.cloud.oracle.com/en-us/iaas/Content/API/SDKDocs/typescriptsdkgettingstarted.htm#Getting_Started
Example :
[DEFAULT]
user=ocid1.user.oc1..<your_unique_id>
fingerprint=<your_fingerprint>
key_file=~/.oci/oci_api_key.pem
tenancy=ocid1.tenancy.oc1..<your_unique_id>
customCompartmentId=ocid1.compartment.oc1..<your_unique_id>
How to create OCI API signing key
4. Once the credentials are set and inputs are provided in upload-config.json. We are all set to run the util.
This will create a bucket with visibility public and publicAccessType = ObjectReadWithoutList.
It will upload all the files in the user-selected folder (mentioned as webdir in upload-config.json) to the bucket.
The util will print the url of the index file. This will be the website homepage url.
Custom Domain
We can configure this as a http redirect or url forwarding to use the custom domain.